This blog post will cover how to drop messages in IBM MQ using dotnet core. The post is based on the other awesome post from dotnet cookbook which I recomend you to read! The objective here is to present an overview of the code, how to use the tracing logs for troubleshooting connections and how to add certificates to perform TLS connections, all of these using the library ibm-mq-client available in Nuget.
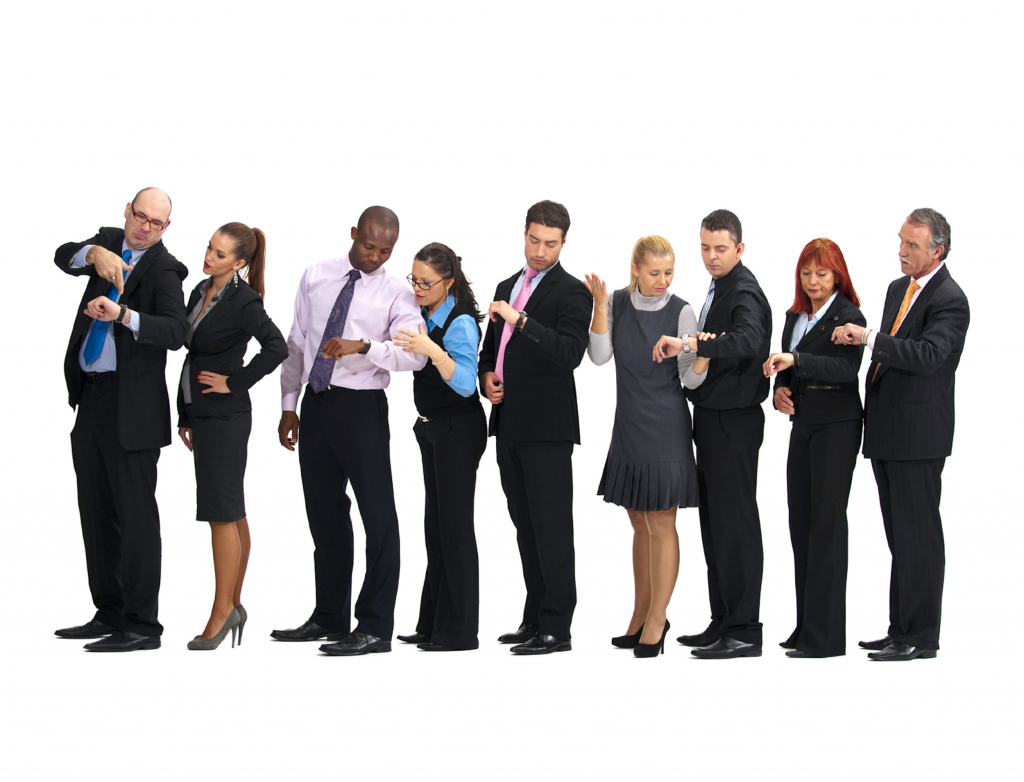
The code to drop messages in IBM MQ
The code basically relies on some parameters for hostname, channel, queue manager, queue and port. Also there are some checks based on the name of those parameters to decide if they contain the pattern “.TLS” in the string. But if in your case you always use TLS on your connections, you can just remove that condition and let the SSL_CIPHER_SUITE_PROPERTY and SSL_CIPHER_SPEC_PROPERTY always available:
Using this code you should be able to drop messages, connect to the queues and achieve the result you want!
But if something goes wrong, you can always trace the messages made available by the library.
Tracing the messages sent to IBM MQ
If you want to debug the logs inside the IBM MQ, you can achieve that by enabling some Environment variables on Windows or Linux. It depends on your stack, but you can add those same variables to your Cloud environment too.
The environment variables necessary are:
MQDOTNET_TRACE_ON (default value is -1 or 0, but it can set for anything more than 0 for more detailed information).
- 0: Stops tracing – this is the default value.
- 1: Starts tracing with lesser details.
- 2: Starts tracing with full details – recommended.
MQTRACEPATH (default value is empty, so it probably will generate the logs inside the folder where your application is running).
MQERRORPATH (default value is empty so it probably will generate the logs inside the folder where your application is running).
The script below will set the environment variables in Windows.
# for Windows you must run CMD/POWERSHELL as ADMIN setx /M MQDOTNET_TRACE_ON "2" New-Item -ItemType Directory -Force -Path C:\temp-logs setx /M MQTRACEPATH "C:\temp-logs" setx /M MQERRORPATH "C:\temp-logs"
Once you initialize those variables, everytime your code tries to connect to an IBM MQ and drop messages there, a file containing the logs will be generated on the path you configured. This can be useful to understand when a connection doesn’t go well.
TLS connections to IBM MQ in .NET Core
In my recent scenario, IBM MQ was configured to use TLS 1.2. If that’s your case, you are going to need a certificate to ensure the connection is secure and that you can drop the messages and connect to the Queue Managers.
In Windows you can reference your local Certificate Store for User or System just like that inside the code:
Hashtable connectionProperties = new Hashtable();
connectionProperties.Add(MQC.SSL_CERT_STORE_PROPERTY, "*USER");
// OR
connectionProperties.Add(MQC.SSL_CERT_STORE_PROPERTY, "*SYSTEM");
But in Linux you have three options:
- Set an specific folder as your Certificate Store using the Enviroment variable MQSSLKEYR that is another IBM MQ dedicate environment variable;
- Define a connection property named MQC.SSL_CERT_STORE_PROPERTY in you Hashset;
- Use the default one which Linux uses (I suggest to go with this one).
Once you define any of the stores, you will need the Certificates. The IBM MQ certificates that you are going to need are the Root certificate and the Intermediate certificate. Please take a look at IBM documentation.
During runtime you can add the certificates and if you go by the defualt one available in Linux, you won’t need to set a environment variable for the Certificate store, neither in the hashset containing Connection Properties. I will always suggest to stick with the default one because the logs will be very straightforward like this:
0000014B 16:51:43.571557 15.334 Created an instance of SSLStreams
0000014C 16:51:43.571604 15.334 Setting current certificate store as 'Computer'
0000014D 16:51:43.571631 15.334 Linux so use My & CurrentUser
0000014E 16:51:43.571652 15.334 Created store object to access certificates
0000014F 16:51:43.571699 15.334 Opened store
00000150 16:51:43.571735 15.334 Accessing certificate - ibmwebspheremqvcap
00000151 16:51:43.576864 15.334 Number of certificates in the store:2
00000152 16:51:43.577017 15.334 TLS12 supported - True
00000153 16:51:43.578221 15.334 Setting SslProtol as Tls12
00000154 16:51:43.578278 15.334 Starting SSL Authentication
For adding the certificates for any Operation System, you can simply add the certificates to the Personal store of the Current User using this code:
This way it’s going to work and you will be able to drop messages in IBM MQ using dotnet core.
References:
dotnet cookbook
IBM.com
Thank you very much Wiliam, helped me a lot
You are welcome, Lucas! 😀
Thank you, but how can I connect to queue manager using TLS_RSA_WITH_AES_256_CBC_SHA256
Well, did you try changing cipherSpec and cipherSuite for your pattern?
Take a look at this post related to different ciphers supported: https://www.ibm.com/support/knowledgecenter/SSFKSJ_7.5.0/com.ibm.mq.dev.doc/q031290_.htm
Hey William
I am curious what version of amqmdnetstd you might be using? I am running 9.1.2 and my logs look quite different. Most interesting is this “Linux so use My & CurrentUser” from yours, mine is still saying “Windows/Mac so use My Store & CurrentStore”. I have my RunTimeIdentifier set to Linux 64, did you do anything else to get it to act differently? Under Windows the Cert was located by looking for one with a friendly name that matched the ibmwebspheremq+app pool id, this is not supported in Linux from what I am understanding so my certificate location is failing.
Hey William
I am curious what version of amqmdnetstd you might be using? I am running 9.1.2 and my logs look quite different. Most interesting is this “Linux so use My & CurrentUser” from yours, mine is still saying “Windows/Mac so use My Store & CurrentStore”. I have my RunTimeIdentifier set to Linux 64, did you do anything else to get it to act differently? Under Windows the Cert was located by looking for one with a friendly name that matched the ibmwebspheremq+app pool id, this is not supported in Linux from what I am understanding so my certificate location is failing.
Any thoughts would be much appreciated
Patrick
Hey William
I am curious what version of amqmdnetstd you might be using? I am running 9.1.2 and my logs look quite different. Most interesting is this “Linux so use My & CurrentUser” from yours, mine is still saying “Windows/Mac so use My Store & CurrentStore”. I have my RunTimeIdentifier set to Linux 64, did you do anything else to get it to act differently? Under Windows the Cert was located by looking for one with a friendly name that matched the ibmwebspheremq+app pool id, this is not supported in Linux from what I am understanding so my certificate location is failing.
Thanks,
Patrick